Category: Robert’s Blog
Revisiting Old Music: Mission Accomplished
Well, it only took me twelve years. I have finally finished my quest to re-listen to my entire CD collection. This really didn’t need to take more than decade, but in the middle of the process I decided to start reviewing each CD individually. After a while the thought of having to type a new review if I listened to a disc would discourage me from continuing. I finally gave up somewhere in compilations. The world doesn’t need to know that each of the eight or so Back from the Grave compilations sounds pretty much like all the other ones. On top of that, there was an excursion into my vinyl collection in which I ripped all my 45s and then started in on my LPs too. Who knew listening to music was such hard work.
My final thoughts are that I like most of the music that I have bought. Who’da thunk it? At the very bottom there a few stinkers which I keep mainly because either they are my wife’s (A Very Special Christmas, Lou Reed) or they have maybe one song that’s worth listening to (Planet P, Lambada, Bob Mould solo crap). I also have a box of freebies that bands have given me and junky Lumpen Media compilations which are mostly crap but I need to keep for archival reasons.
CDs are getting a bad rap these days. Other than a good old fashioned punk rock 45, I still think CD is my preferred format for physical media music. If anyone tells you vinyl sounds better, they are lying. I only wish that instead of jewel cases they were packaged in thinner sleeves. A little known benefit of CDs is that they don’t really appreciate in value which means they are super cheap to buy used. Often cheaper than MP3 albums (Discogs is a great way of building up a killer rare CD collection).
Three More Martian Law Videos
Here are three more videos of my Martian Law songs. These were all created in FL Studio.
“Natural Kitty” Official Video
This is a track from my second computer music release called, Upgrade Downgrade (2001). The music was created in MadTracker 2 on a Windows 98 PC. The voice was from AnalogX’s SayIt software and most of the samples were probably found online somewhere.
“March of the Mustard King” Official Video
20 years ago this month I released an album’s worth of computer music. If you weren’t one of the lucky 3 people who got a CD, this is what you missed. Last night I made an *official video* for the song “March of the Mustard King.” This was pre-Garage Band. Back when making songs on a computer was like watching the code of the Matrix scroll by. Music by nerd(s), for nerds.
This is a track from my first album of computer music called, “The Exciting Sounds of a Compaq P133” (1999). The music was created in FastTracker ][ on a Windows 98 PC. This video is showing the excellent FastTracker ][ Clone from 16-bits.org.
How to Import Books to an iPad 1 (Without iTunes)
Update (June 2020): All of the sudden, iTunes started supporting books on the iPad 1 again so this article is mostly moot. I leave it here for posterity.
Apple has a history of abandoning users whenever they introduce a new product to the market. This wasn’t always the case. The Apple IIgs, despite its 16-bit architecture, allowed for most of the old 8-bit Apple ][ software to run on the new system. However, since the introduction of OSX, the attitude has been, “Deal with it old-timers. We know what’s best for you.”
I am the proud owner of a first generation iPad 1. I use it to play music and read eBooks. It performs these two tasks as well as any modern iPad or iPhone does. Alas, in their attempt streamline the software, Apple removed to ability to transfer PDFs and eBooks to an iPad 1 from iTunes. The entire Books section has been removed from the product with the expectation that iPad users will now get their content from the cloud. The problem is that iCloud, Dropbox and other cloud storage systems no longer work on an iPad 1.
My attempts to Google a solution have had mixed results. Apple is no help. The user help forums are filled with bad advice. In my frustration I have figured out a relatively simple way to get files, especially books, to your iPad 1. In a nutshell, we are going to create a web page containing links to all your books and then, assuming your computer and ipad are on the same local wi-fi network, browse to that web site on our iPad to download the files to iBooks.
The only catch here is that the process requires you to download a piece of software to your Mac or PC. The application is called Prepros. It is free to download and use from Prepros.io. It is an excellent piece of software that is normally a tool used for Web development to pre-process code and make it ready for a Web page. But we are going to use it as our file server.
Step 1: Download, Install and Run Prepros
If installing an application is too complicated for you, maybe you should stick to paper books.
Step 2: Organize Files for Transfer
Put any files that you want to transfer to your iPad in a single folder. In my example I have put a bunch of PDFs into a folder on my desktop called “GOG.com Manuals.” Here’s my folder next to the open Prepros app window:
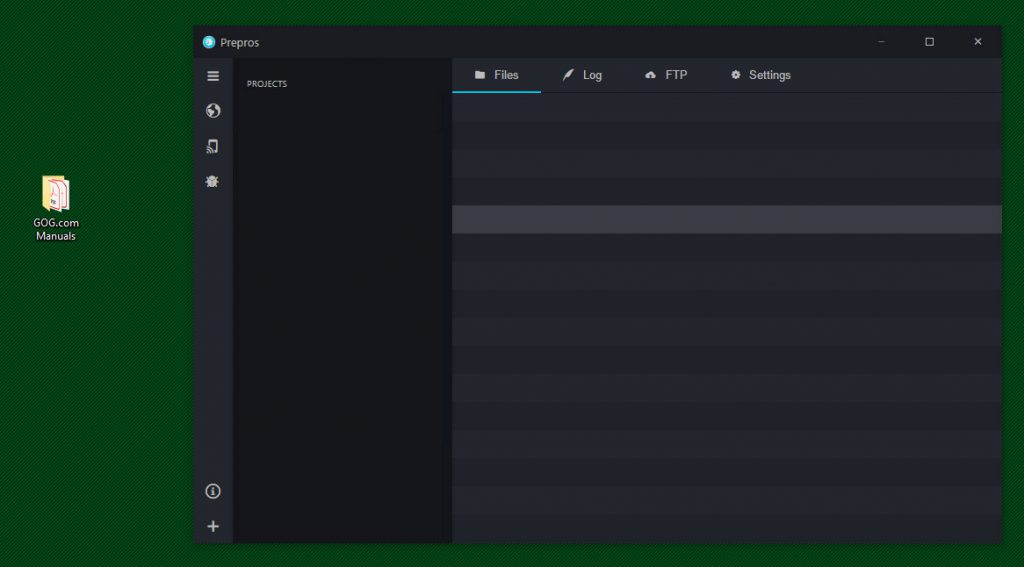
Step 3: Drag and Drop your Folder into Prepros
Drag your folder into the Prepros window and drop it into the “Projects” panel.
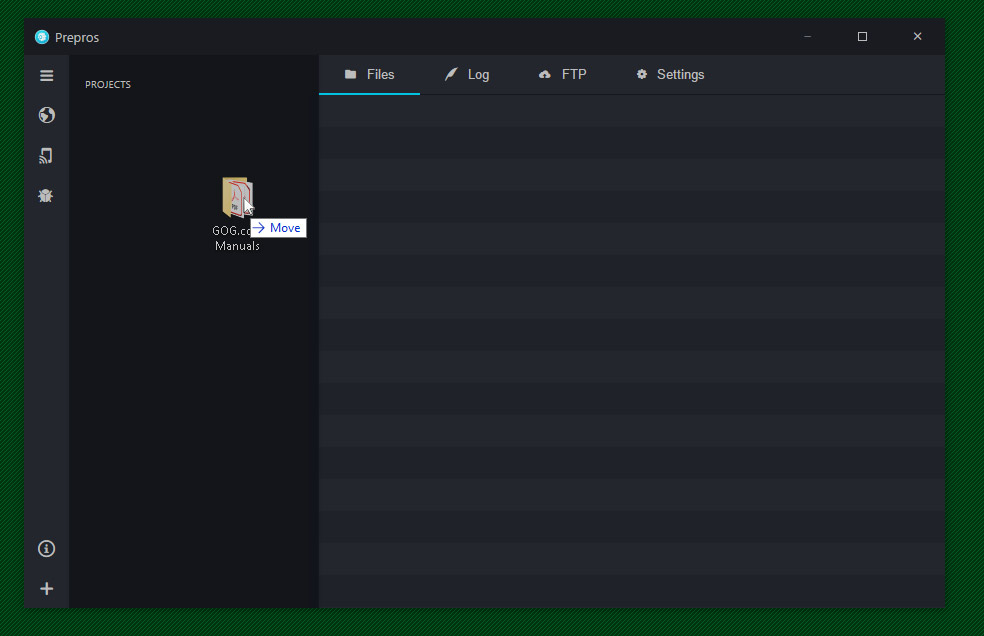
Your folder will now appear as a “Project” with any files from the folder listed in the right panel under “Files.”
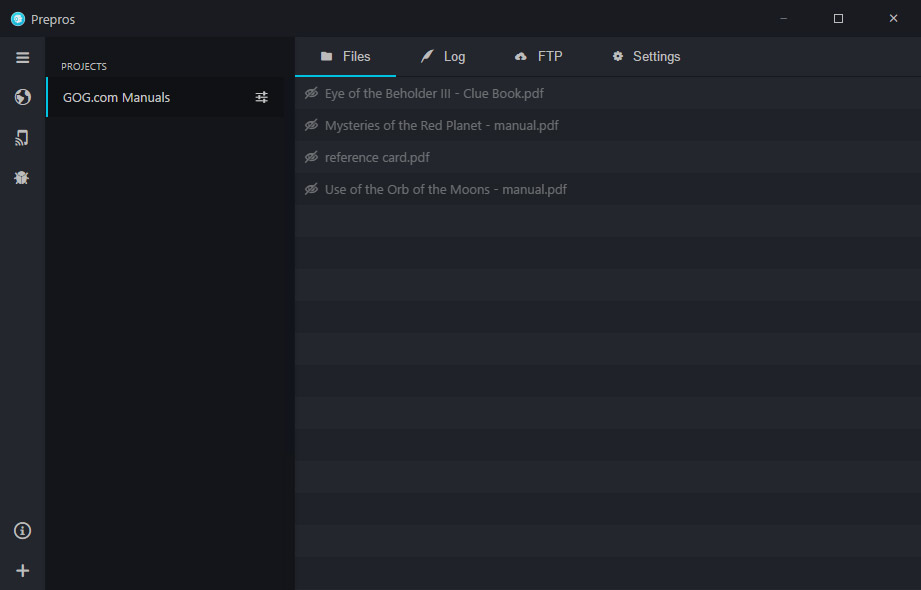
Step 4: Start the File Server
We are now going to start a local network file server by clicking the Network Preview icon (looks like a Wi-fi/Phone) on the left side of the window:
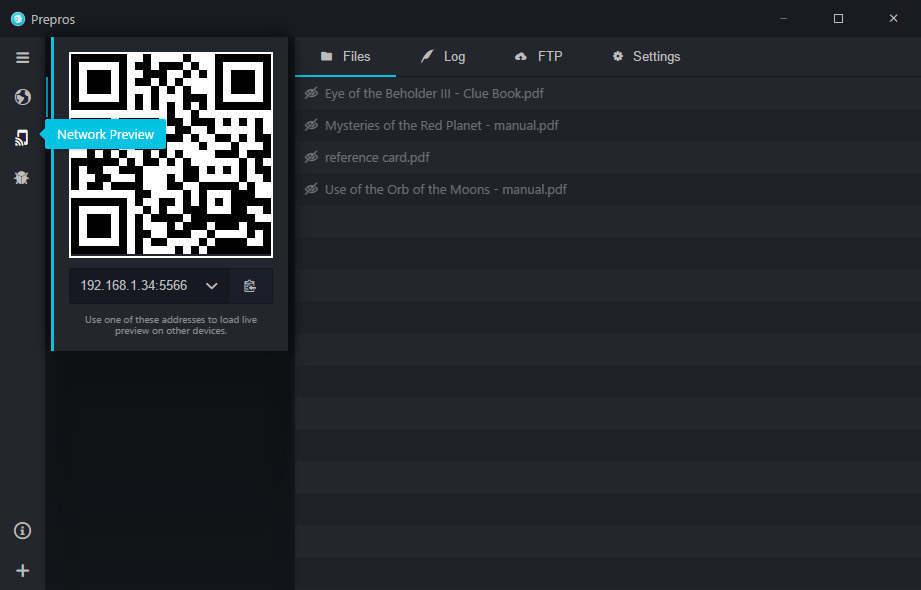
If an iPad 1 had a camera we could scan the big QR code, but for us old-timers, an IP address is provided in the popup. Note the number in the box. In the above example the IP address is 192.168.1.34:5566
Step 5: Type the IP Address into Safari on your iPad 1
Turn on your (wi-fi connected) iPad, open Safari and enter the IP address into the location bar. Your Prepros file server will appear as a Web page:
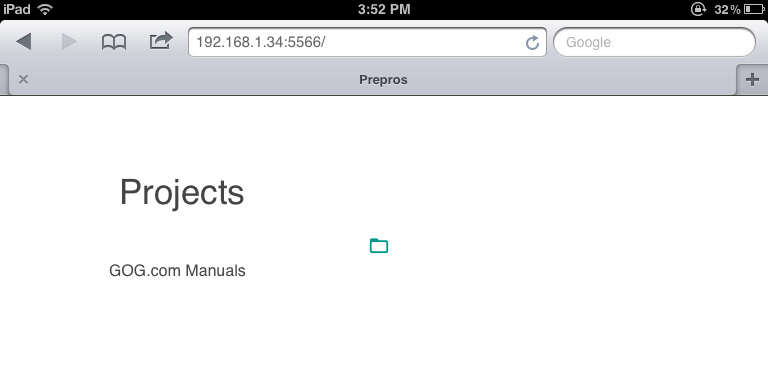
Tap on the appropriate folder to see your list files. In this example, there is only my “GOG.com Manuals” directory.
Step 6: Tap on the File you Want to Transfer to iBooks
Your files from your PC or Mac should now be visible (there will also be a file called prepros-6.config, ignore that). Tap on the PDF you want to open the PDF in Safari:
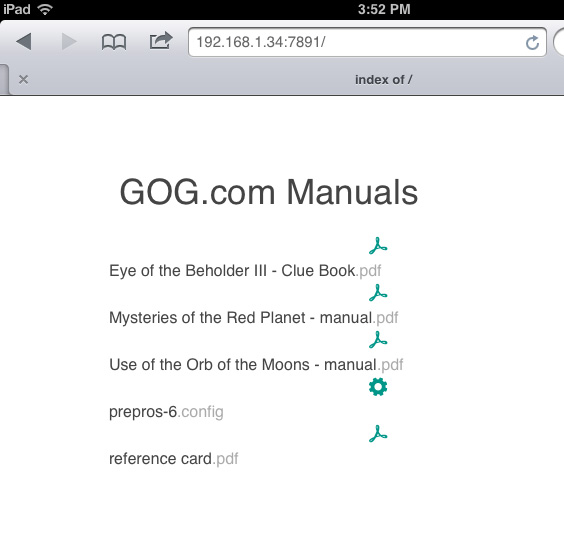
Step 7: Tap the Open in iBooks Button
The PDF will render in the Safari window. A couple of buttons will also appear at the top of the PDF which will allow you to transfer the PDF into your iBooks library. If the buttons aren’t visible tap the PDF page to make them reappear. Tap the Open in iBooks button:
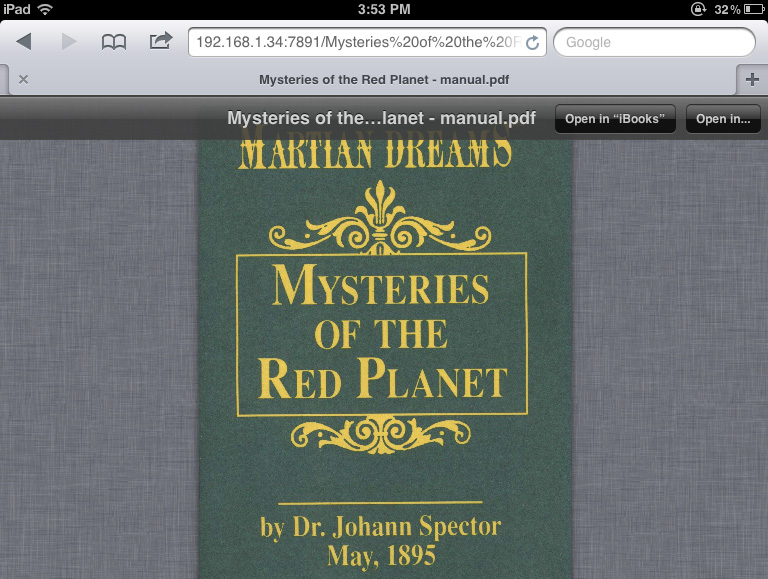
Step 8: Enjoy your eBook on your iPad!
The iPad should automagically switch to iBooks and your PDF/ebook will appear on your shelves. You can keep transferring books, or, if you are done, close out of Safari and exit Prepros on your computer. The book is now permanently on your iPad.
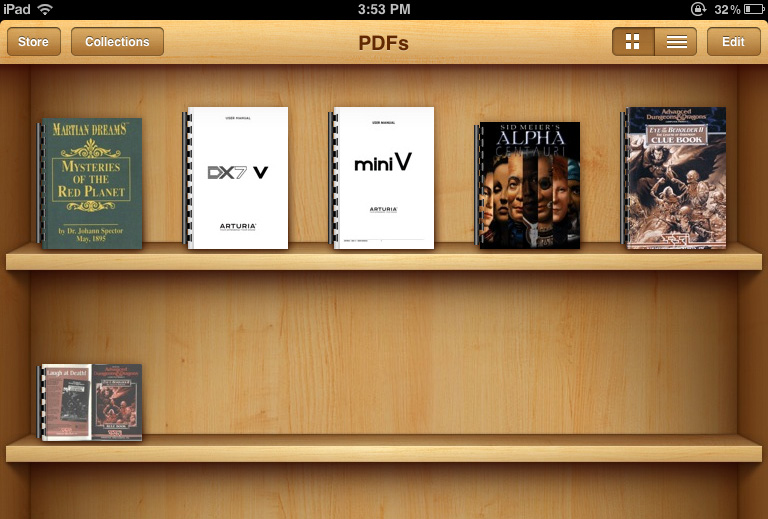
I know this seems like a lot of steps, but I want to be thorough. In this age of command line interfaces, detailed tutorials are hard to come by.
P.S. If you like Prepros, please buy it. It is one of the only shareware programs I have ever bought and I use it every single day as a Web developer.
Goodbye Old Friend
It’s been just about a year since I purchased The Witcher 3 from GOG.com and I have finally finished the DLC content. The Blood and Wine expansion like getting a completely new game world to explore. Worth every penny. But alas, I think it’s time to uninstall this fantastic game. I suppose I could keep going to try to complete all the achievements but my time and SSD space is limited. For now I will patiently await the release of Cyberpunk 2077. On the up-side, I will never be forced to play a boring round of Gwent again.
Apple ][ Graphic Adventure Part V
Now that my memory issues are seemingly under control, let’s take a look at my modifications to the parser. Normally, in these types of graphical adventures the player enters two words in the form of VERB OBJECT
. My interface limits the number of verb choices and allows the player to enter a verb with a single keystroke.
In Applesoft you can prompt for user input in two ways. First there is INPUT A$
which will display a question mark on the screen and await user input followed by a RETURN. That user response then fills the variable A$. Similarly there is GET A$
which also displays a question mark but GET
will only accept a single keypress as user input. My main problem with both of these is an aesthetic one: that darn question mark.
The solution is to write your own input routine leveraging machine code routines via PEEKs
and POKEs
. To do this, first I simulate a cursor by placing a flashing underscore character at the bottom of the screen.
101 VTAB 24 : HTAB 1 : CALL -868 : PRINT ":"; : FLASH : PRINT "_"; : NORMAL : GOSUB 55
A lot is going on in this line. The VTAB
and HTAB
commands position the screen cursor at line 24 and character 1. CALL -868
is a special machine code call that clears that single line of text. Now that we have an empty line we type a colon and then a flashing underscore. The result looks like this:
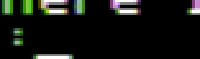
This looks like a user input prompt, but at this point it does nothing. The magic happens at the subroutine which is GOSUB’d at the end of that line.
55 KEY = PEEK (49152) : IF KEY < 128 THEN 55
56 IF KEY > 224 AND KEY < 251 THEN KEY = KEY - 32 : REM UPPERCASE
57 POKE 49168,0 : BUZZ = PEEK (49200) : RETURN
In line 55 we are creating a variable KEY and assigning to it the contents of memory location 49,152 to it ($C000 for you hex-heads). Turns out location 49,152 will read the keyboard and return the ASCII value of the currently pressed key. If that value is a character then we break out of the loop and go to line 56.
Line 56 insures that, if the ASCII value of the key denotes a lowercase key, it is converted to uppercase by shifting the ASCII value. POKE 49168,0
clears the keyboard buffer so that the PEEK in 55 will work next time around and not just register the same value. Finally, that BUZZ = PEEK (49200)
bit triggers a speaker click so that the player’s keystroke has and audible sound.
When we return to the main game loop we now have a variable KEY which contains an ASCII value of the key pressed. I can then branch the program based on this value. I can also test if it’s a RETURN keypress and then toggle text display. Later in my program I can concatenate keypresses into a single string value by returning to that subroutine again and again until a return press is detected. That’s how I collect the OBJECT half of the VERB OBJECT pair.
Apple ][ Graphic Adventure Part IV
Previously I discussed the overall structure of my soon-to-be hit adventure game. Well, last night was a milestone. I managed to write an Applesoft program so epic that it overwrote the high-resolution graphics page. Compared to other programs I have seen, mine isn’t that huge. Around 250 lines isn’t that huge, right? Transylvania clocks in at 464 lines.
I think the issue is the number of arrays that I am defining. For now I think I have a fix. I have set LOMEM: 24576
at the top of my program. Supposedly, this will force the interpreter to define variables in a memory location after the hi-res pages. We shall see.
In any event, the game is back and running again. And the text screen now has some text formatting enhancements:
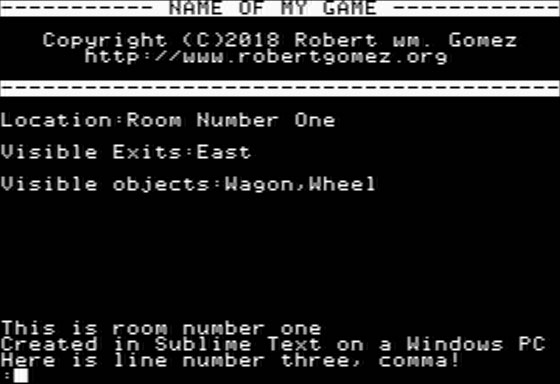
One of the cooler things I have implemented is this text screen. If at any prompt you hit RETURN you turn off the hi-res graphics and can see this text screen. Here will be some valuable game info included the location’s name, exits and any TAKE-able objects. The code for this is rather simple:
58 IF GM = 1 THEN GM = 0 : TEXT : RETURN
59 GM = 1 : CALL -3100 : RETURN
GM is a flag which tracks where you are in graphics mode (1) or text mode (0). CALL -3100
triggers the hi-res graphics screen without erasing its contents. So exciting, right!?!
Apple ][ Graphic Adventure Part III
The previous post in this series explained how to get Graphics Magician images to display from Applesoft. Now, I’d like to go over the structure of the program listed in Write your Own Adventure Programs. The bulk of the program listing consists of the game data including objects, room descriptions, verbs and state flags. Most of the remaining code is comprised of a series of conditions that check how the player’s actions affect the objects in the game world.
Verbs
Haunted House used a simple, two-word input parser: VERB NOUN. But I wanted this new game to simplify the number of verb choices in the same way the LucasArts adventures streamlined the interface of Sierra-style adventure games. The player will be limited to around a dozen verbs that are entered with a single keystroke.

The verb list is largely based on the options in Monkey Island. PUSH and PULL have been combined into MOVE. To move you must hit Go then enter either North, South, East, West, Up or Down. This is a little annoying, but there are only so many letters in on the keyboard and I needed that D, U and S elsewhere. Other commands require you to hit the keystroke, then type out an object NOUN and then hit Return. “Guess the verb” will no longer be an issue… welcome to 21st century “guess the noun” technology!
Each verb then get’s its own subroutine which contains the logic that triggers the various game actions (or provides a default message if nothing special happens). By assigning a number value VB to each verb, I can use the following to branch to the various subroutines: ON VB GOSUB 1000,600,800,850, ...
Game Data
The game data is set in the program by assigning strings and numbers to several arrays. In Applesoft you need to declare the size of an array by dimensioning it with the DIM
command. For the rooms I will set the size of the rooms array to the number of rooms RM by declaring DIM RM$(RM)
. Then, near the start of my program I read data into the array by using GOSUB
to a loop like this:
5000 DATA "Room description 1","Room Description 2", [...]
5005 FOR I = 1 to RM : READ RM$(I) : NEXT
5010 RETURN
The DATA
can be listed anywhere in the code and it’s important to make sure that there are exactly as many data strings as READ
commands. Otherwise, you might get OUT OF DATA
errors.
This method of declaring rooms and object will eventually make your Applesoft program very long and hard to edit. I was pretty sure that I could figure out a way to read the data in from an external text file. But more on that later.